The Floating Action Button (FAB) is a prominent UI element that represents a primary action in an app. It floats above the content and provides easy access to the most important functionality. The FAB is often used for actions like adding a new item, composing a message, or initiating a crucial operation.
To create a Floating Action Button in Jetpack Compose, we utilize the FloatingActionButton
component from the Material Design library. And in this post, we will use the Material 3 library.
Key Points about FAB:
- FAB should be used for the most common or important action on a screen.
- Icons in a FAB must be clear and understandable because these buttons do not have a text label.
- The FAB should persist on the screen when content is scrolling.
Floating Action buttons in jetpack compose have three sizes:
- FAB
- Small FAB
- Large FAB
1. Floating Action Button
FloatingActionButton(onClick = { /*TODO*/ }) {
IconButton(onClick = { /*TODO*/ }) {
Icon(imageVector = Icons.Outlined.Edit, contentDescription = null)
}
}

FloatingActionButton
function has many parameters but only two are compulsory and others are optional. Compulsory are:
onClick
: It is called when this FAB is clickedcontent
: This parameter defines a lambda that contains the content of this FAB, typically an Icon.
Customizing the FAB
Jetpack Compose provides several customization options for the Floating Action Button. We can modify its appearance, position, and behaviour to align with our app’s design language. Some common customizations include:
- shape
- elevation
- container color
- content color
Let’s see them with an example:
1. Shape
Note: You can use the modifier to manipulate the position of the floating action button. We will not discuss modifiers here. Check out our post on modifiers if you are not aware of them.
1. Circle
FloatingActionButton(
onClick = { /*TODO*/ },
shape = CircleShape
){...} // code is same as above in curly brackets

2. Rectangle
FloatingActionButton(
onClick = { /*TODO*/ },
shape = RectangleShape
){...} // code is same as above in curly brackets

2. Color
In this section, we will change the color of fab and its content.
1. Container color
This parameter of FloatingActionButton
function sets color to the background of the FAB.
FloatingActionButton(
onClick = { /*TODO*/ },
containerColor = Color.Yellow
){...}

2. Content Color
This parameter sets the color to the content of the FAB
FloatingActionButton(
onClick = { /*TODO*/ },
containerColor = Color.Yellow,
contentColor = Color.Magenta
){...}

3. Elevation
This parameter is used to resolve the elevation for FAB in different states. This controls the size of the shadow below the FAB.
FloatingActionButton(
onClick = { /*TODO*/ },
elevation = FloatingActionButtonDefaults.elevation(16.dp)
)

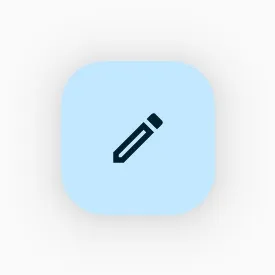

2. Small Floating Action Button
SmallFloatingActionButton(onClick = { /*TODO*/ },) {
IconButton(onClick = { /*TODO*/ }) {
Icon(imageVector = Icons.Outlined.Edit,
contentDescription = null)
}
}


3. Large Floating Action Button
LargeFloatingActionButton(onClick = { /*TODO*/ },) {
IconButton(onClick = { /*TODO*/ }) {
Icon(imageVector = Icons.Outlined.Edit,
contentDescription = null)
}
}


Note: For styling of these small and large floating action buttons, please refer to the styling of simple floating action as all parameters are the same in all the FABs.
The Floating Action Button is a crucial component in modern Android app design, and with Jetpack Compose, creating and customizing FABs has become more straightforward.
Some Useful links
Happy Coding 🙂