Jetpack Compose Time Input helps users enter a specific time. We have already discussed the Time Picker component of the jetpack compose in our last article. Time Input does the same thing as Time Picker, but the user must manually enter the specific time of the day whereas Time Picker helps the user select the specific time.
Usages of Time Input
Time Input is used in all types of applications. We are providing some categories:
- Appointment Scheduling
- Task Management
- Event Planning
- Reminder and Alarms
- Booking and Reservations
- Health and Fitness Tracking
Numerous categories still await mention.
Prerequisites:
Note: We use the latest version of Material 3 APIs and the BOM Version as given below:
implementation(platform("androidx.compose:compose-bom:2023.10.01"))
Please change your BOM version in the build.gradle.kts(Module:app)
to the given version or the latest version.
Time Input
A time input is a user interface (UI) element in the jetpack compose that helps the user enter a specific time. It helps the user enter the time accurately. Let’s see the default method declaration in the Android APIs:
fun TimeInput(
state: TimePickerState,
modifier: Modifier = Modifier,
colors: TimePickerColors = TimePickerDefaults.colors(),
)
Explanation:
- state – This manages the state of the time input element.
- modifier – It helps in applying different – different modifiers to our time input.
- colors – As the name suggests, help in decorating the time input.
Note: The only state parameter is mandatory means modifier and colors are optional.
Introducing Jetpack Compose Time Input
val myState = rememberTimePickerState(9, 15, false)
Column(
horizontalAlignment = Alignment.CenterHorizontally,
verticalArrangement = Arrangement.Center
) {
TimeInput(state = myState)
Text(text = "Time is ${myState.hour} : ${myState.minute}")
}
First of all, we get the state from the rememberTimePickerState
method so that our time input component can survive the recomposition. This takes 3 mandatory parameters with some default values:
- initialHour – This represents the hour hand of the clock. The default value is 0 and ranges from 0 to 23.
- InitialMinute – It is the minute hand of the clock. The default value is 0 and ranges from 0 to 59.
- is24Hour – This parameter expects a Boolean value where false means 12-hour format and true means 24-hour format. The default value is picked from the system settings.
In the TimeInput
function, we simply pass our mystate
variable as argument to state
parameter. And lastly, Text composable shows the user entered time taken from the mystate
.
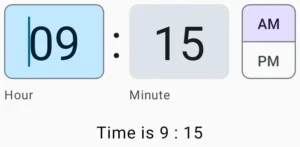
Note: if, we change the is24Hour
parameter value to true
in the rememberTimePickerState method then the TimeInput UI element will not have AM/PM.
val myState = rememberTimePickerState(9, 15, true)
// remaining code is same
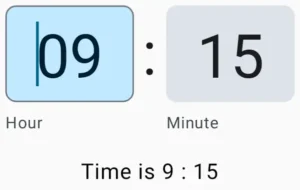
Customizing Time Input
In this section, we use colors
parameter of TimeInput
method to give some colours.
TimeInput(
state = myState,
colors = TimePickerDefaults.colors(
timeSelectorSelectedContainerColor = Color.Green,
timeSelectorSelectedContentColor = Color.Magenta,
timeSelectorUnselectedContainerColor = Color.LightGray,
timeSelectorUnselectedContentColor = Color.Red,
periodSelectorBorderColor = Color.Yellow,
periodSelectorSelectedContainerColor = Color.Cyan,
periodSelectorSelectedContentColor = Color.Blue,
periodSelectorUnselectedContainerColor = Color.Gray,
periodSelectorUnselectedContentColor = Color.Red
)
)
The code written above is self-explainatory.
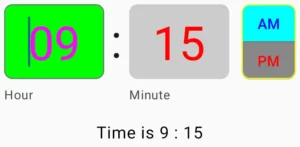
Well, that’s all for this article. I hope this article will add value to the users. Please comment on your questions and problems.
Happy composing 🙂