A “lazy vertical grid” refers to a UI layout where items are arranged in a grid format, but the grid is created and rendered lazily, meaning that only the visible items are rendered at a given time. This approach is often used to optimize performance when dealing with large datasets or dynamically changing content. In this blog post, we’ll delve into LazyVerticalGrid and discover how it simplifies grid-based UI implementation in Jetpack Compose.
Understanding LazyVerticalGrid
LazyVerticalGrid is a compositional component in Jetpack Compose that allows developers to create a grid-based layout where items are lazily loaded. This laziness ensures that only the visible items are rendered, resulting in improved performance and reduced memory consumption.
1. Simple LazyVerticalGrid
First of all, we create a list of country names.
val listOfName = listOf("India", "China", "Pakistan", "Japan", "USA", "UK", "Canada", "UAE")
Now, we call LazyVerticalGrid
function.
LazyVerticalGrid(columns = GridCells.Fixed(2)) { }
columns
– This parameter describes the count and the size of the grid’s columns.
Note: We use GridCells class to describe the number of grids because this class describes the count and the sizes of columns in vertical grids, or rows in horizontal grids
LazyVerticalGrid(columns = GridCells.Fixed(2)) {
items(listOfName) {
Text(text = it)
}
}
Now inside the lambda, we call the items
function which takes the data list as a parameter. And in the lambda body, the Text composable function is called for every item in the list and it
refers to the value of the current index of the list.
Output:

2. Advance LazyVerticalGrid
In this section, we will create a simple grid list of cats.
1. Data Class
A data class is a class that holds the data. Now, we create a class that holds the name and image of the Cat.
data class Cat(
@DrawableRes val imageResId: Int,
@StringRes val stringResId: Int
)
In the above code, the variable imageResId
and stringResId
are annotated with @
and DrawableRes
@StringRes
.
stringResId
– It represents an ID for the cat name text stored in the string resource.imageResId
– it represents an ID for the cat image stored in the drawable resource.
Note: Never use strings directly in the code, always store them in a string resource file (strings.xml)
located at /res/values/
2. Required Files
First of all, add cat names in the strings.xml
file.
<string name="cat_1">Cat 1</string>
<string name="cat_2">Cat 2</string>
<string name="cat_3">Cat 3</string>
<string name="cat_4">Cat 4</string>
<string name="cat_5">Cat 5</string>
<string name="cat_6">Cat 6</string>
<string name="cat_7">Cat 7</string>
<string name="cat_8">Cat 8</string>
<string name="cat_9">Cat 9</string>
Now add images in the /res/drawable
folder.

3. Cat List
Now, we create a function that will return a list of cat data class.
fun catList(): List<Cat> {
return listOf(
Cat(R.drawable.cat_1, R.string.cat_1),
Cat(R.drawable.cat_2, R.string.cat_2),
Cat(R.drawable.cat_3, R.string.cat_3),
Cat(R.drawable.cat_4, R.string.cat_4),
Cat(R.drawable.cat_5, R.string.cat_5),
Cat(R.drawable.cat_6, R.string.cat_6),
Cat(R.drawable.cat_7, R.string.cat_7),
Cat(R.drawable.cat_8, R.string.cat_8),
Cat(R.drawable.cat_9, R.string.cat_9)
)
}
4. Cat Card
Now, we create a function that will present the cat image and name horizontally on a Card.
@Composable
fun CatCard(cat: Cat, modifier: Modifier) {
Card(modifier = modifier) {
Row(
modifier = Modifier.padding(8.dp),
verticalAlignment = Alignment.CenterVertically,
horizontalArrangement = Arrangement.spacedBy(16.dp)
) {
Image(
painter = painterResource(id = cat.imageResId),
contentDescription = stringResource(id = cat.stringResId),
modifier = Modifier
.size(100.dp)
.clip(CircleShape),
contentScale = ContentScale.Crop
)
Text(text = stringResource(id = cat.stringResId))
}
}
}

stringResource
– This function fetches the string from strings.xml
by taking id as a parameter.
5. LazyVerticalGrid
Now, we create a function that takes two parameters, one for the cat list and one for the modifier.
@Composable
fun LazyGridExample(listOfCat: List<Cat>, modifier: Modifier) {
LazyVerticalGrid(columns = GridCells.Adaptive(150.dp)) {
items(listOfCat) { cat ->
CatCard(cat = cat, modifier = modifier.padding(4.dp))
}
}
}
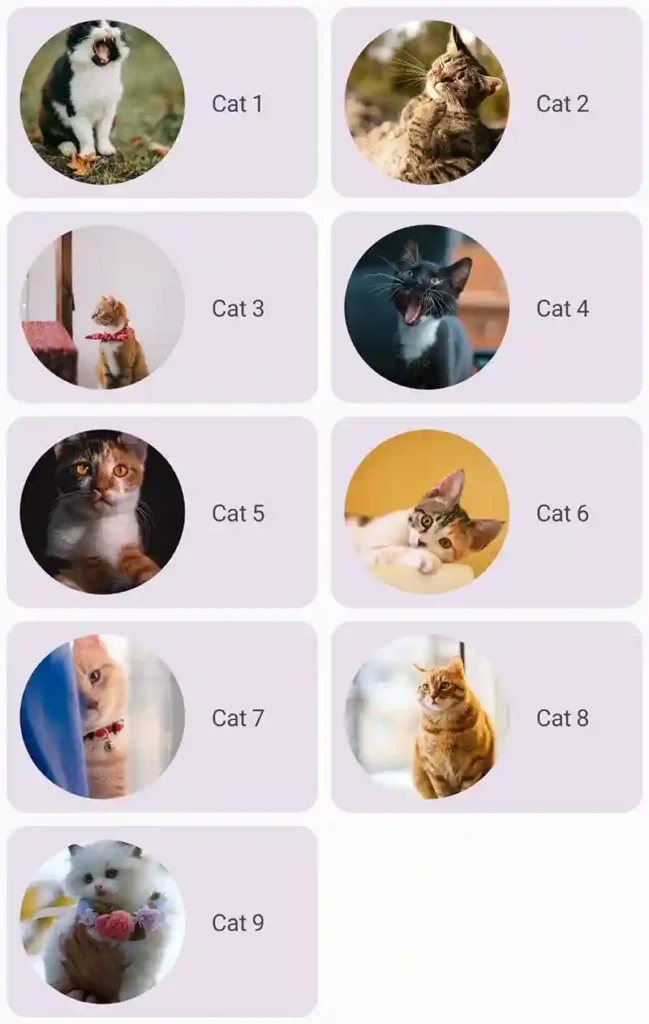
GridCells.Adaptive(150.dp)
– It defines a grid with as many rows or columns as possible on the condition that every cell has at least minSize space and all extra space is distributed evenly. In the code above, we used 150 DP, which assures that every card will have a minimum of 150 dp.
In items()
function lambda body cat
parameter represents one cat item from the catList
.
Note: LazyVerticalGrid function takes many parameters like contentPadding, flingBehavior etc. We will discuss them in future posts.