In modern app design, swipe-to-dismiss functionality provides a seamless user experience by allowing users to effortlessly remove items or screens from view. Jetpack Compose, the declarative UI toolkit for Android, offers a straightforward way to implement swipe-to-dismiss interactions. In this blog post, we will explore how to integrate swipe-to-dismiss in Jetpack Compose and create an intuitive user interface.
Prerequisites
- The card in Jetpack Compose
- Basic Kotlin
- Update Material 3 library to the latest version
androidx.compose.material3:material3-android:1.2.0-alpha03
Swipe-to-Dismiss
First of all, we create a variable name dismissSate
using rememberDismissState
function that will hold the state for the SwipetoDismiss function.
val dismissState = rememberDismissState()
Now we call SwipeToDismiss
function which is pre-built in jetpack compose.
SwipeToDismiss(
state = ,
background = ,
dismissContent =
)
As you can see in the above code, this function has three compulsory parameters. let’s explore these:
state
– The state of this component
background
– A composable that is stacked behind the content and is exposed when the content is swiped. We can use the state
to have different backgrounds.
dismissContent
– The content that can be dismissed.
SwipeToDismiss(
state = dismissState,
)
We set dismissState
, which was defined earlier, as the state of SwiptoDismiss.
SwipeToDismiss(
state = dismissState,
background = {
val color by animateColorAsState(
when (dismissState.targetValue) {
DismissValue.Default -> Color.Yellow
DismissValue.DismissedToEnd -> Color.Green
DismissValue.DismissedToStart -> Color.Red
}
)
Box(
modifier = Modifier
.fillMaxSize()
.background(color = color)
)
}
)
In the above code, we defined a color using animateColorAsState
function which animates the content when the target value change. We will discuss this in detail in the post of animations. And we defined a when state to pick a colour on the basis of dismiss state.
Finally, we create a Box with the max size and give the earlier created color as the background of the Box
.
SwipeToDismiss(
state = dismissState,
background = {
val color by animateColorAsState(
when (dismissState.targetValue) {
DismissValue.Default -> Color.Yellow
DismissValue.DismissedToEnd -> Color.Green
DismissValue.DismissedToStart -> Color.Red
}
)
Box(
modifier = Modifier
.fillMaxSize()
.background(color = color)
)
},
dismissContent = {
CardShower()
}
)
Now in the above code, we passed CardShower()
function as an argument to the dismissContent
parameter. I hope you all know how to use the Card element in Jetpack compose, if you don’t then check out my previous post on Card. The link is given in the prerequisite section. Let’s see the code of CardShower
function.
@Composable
fun CardShower() {
Card(
modifier = Modifier
.fillMaxWidth()
.padding(8.dp)
) {
Column(
verticalArrangement = Arrangement.Center,
modifier = Modifier.padding(8.dp)
) {
Text(
text = "Jetpack Compose",
style = MaterialTheme.typography.titleMedium
)
Text(text = "Android Development")
}
}
}
In the above code, we create two pieces of text in a column to make it vertical and the column goes into Card.
Complete Code Link
Output:
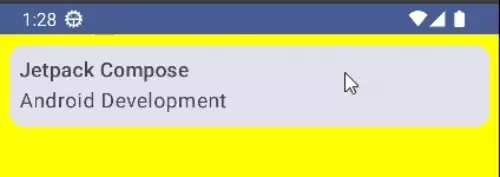
One Side Implementation
If you want to implement swipe action only to one side then you can use an optional parameter (directions) of the SwipeToDismiss function.
directions = setOf(DismissDirection.StartToEnd, DismissDirection.StartToEnd)
In this blog post, we’ve learned how to implement swipe-to-dismiss functionality in Jetpack Compose.
Happy Composing 🙂