Radio buttons are a fundamental UI component that allows users to select a single option from a group of mutually exclusive choices. With the advent of Jetpack Compose, building user interfaces in Android has become more intuitive and declarative. In this blog post, we will delve into the world of radio buttons in Jetpack Compose, exploring how to create and use them effectively in your app.
In Jetpack Compose, the RadioButton
component serves as the equivalent of the traditional radio button in Android’s View-based UI. It provides an easy way to create a group of options where users can select a single choice.
Single Radio Button
To create a radio button in jetpack compose, we will invoke RadioButton
composable function.
val isSelected by remember { mutableStateOf(false) }
RadioButton(
selected = isSelected,
onClick = { /*TODO*/ }
)
isSelected
– It is a variable that holds the current state of the radio button.
selected
– It tells whether the radio button is selected or not.
onClick
– This is called when this radio button is clicked. If null, then the radio button will not be interactable, unless something else handles its input events and updates its state.
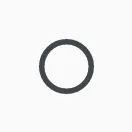
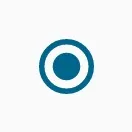
A group of Radio Buttons
First of all, we define a list of options and a state variable to hold the currently selected option.
val radioOptions = listOf("Mango","Banana","Apple","Peach")
val selectedOption by remember { mutableStateOf(radioOptions[0]) }
We build the UI structure using the Column
, Row
and RadioButton
components. We use forEach
, a higher-order function of Kotlin, to iterate over the radioOptions
list and create a RadioButton
for each option.
Column(
modifier = Modifier.padding(8.dp),
verticalArrangement = Arrangement.spacedBy(16.dp)
) {
radioOptions.forEach { fruitName ->
Row(verticalAlignment = Alignment.CenterVertically) {
RadioButton(
selected = (fruitName == selectedOption),
onClick = { selectedOption = fruitName }
)
Text(
text = fruitName,
style = MaterialTheme.typography.bodyMedium,
modifier = Modifier.padding(start = 8.dp)
)
}
}
Text(
buildAnnotatedString {
append("The Selected Value is: ")
withStyle(
style = SpanStyle(
fontWeight = FontWeight.Bold,
color = Color.Magenta)
) {
append(selectedOption)
}
}
)
}
Output:

Let’s delve into the above-written code.
Column
In the column section, we give overall padding of 8 dp and a 16 dp space to all the elements inside the column using Arrangement.spacedBy(16.dp)
.
Row
Inside the row layout, we use Alignment.CenterVertically
to make all the elements of the row layout in the centre. And now we call RadioButton and a Text composable function for each item in the radioOptions
list.
Radio Button
selected
– In this parameter, we compare the currently selected fruit name from the list by the forEach function and the value is in selectedOption
variable which will produce a boolean value(true/false).
onClick
– In this parameter, we set the currently selected fruit name by the forEach function to the selectedOption
variable.
And the remaining code is just holding two texts composable that are easily understandable. The last Text composable function shows the value of the currently selected radio button. And this composable also uses buildAnnotatedString
function to set the colour to only the selected value. If you don’t how to use buildAnnotatedString then check out my post on buildAnnotatedString.
Styling of Radio Buttons
We can disable a radio button from interactivity using enabled
parameter of RadioButton
Composable.
RadioButton(
selected = isSelected,
onClick = { /*TODO*/ },
enabled = false
)
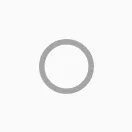
We can change the colour of the radio button using the colors
parameter of RadioButton composable.
RadioButton(
selected = isSelected,
onClick = { /*TODO*/ },
colors = RadioButtonDefaults.colors(
selectedColor = Color.Green,
unselectedColor = Color.Magenta
)
)
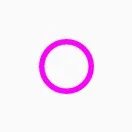
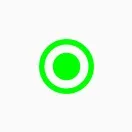
Radio buttons are an essential component for collecting user input in any app. With Jetpack Compose, creating radio button groups has become more streamlined and intuitive. By leveraging the RadioButton
component, you can easily build and customize radio buttons to suit your app’s needs.
Some Useful Links
Happy Composing 🙂
One thought on “Radio Buttons in Jetpack Compose”