Image is a component that is used to show images in UI. There are currently three types of Image composable functions in jetpack compose, for more details visit the official documentation. Before we start, let’s have a look at Image composable function
@Composable
fun Image(
painter: Painter,
contentDescription: String?,
modifier: Modifier = Modifier,
alignment: Alignment = Alignment.Center,
contentScale: ContentScale = ContentScale.Fit,
alpha: Float = DefaultAlpha,
colorFilter: ColorFilter? = null
)
In the image composable function above, only painter
and contentDescription
parameter is compulsory.
- Painter – This parameter helps to draw a painter as an image. We will use
painterResource
to load images fromdrawable
folder. - Content Description – This parameter holds a string that is used by accessibility services to describe what this image represents. It is recommended to use a string from
stringResource
, so that it can be localized.
Image(
painter = painterResource(id = R.drawable.cat),
contentDescription = "cat image"
)
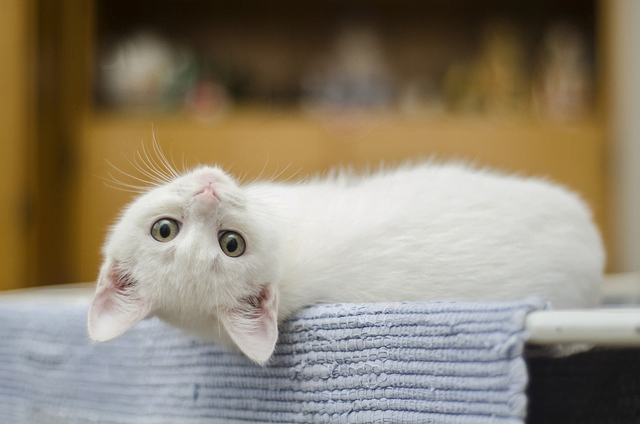
Image Size
We can use modifier
parameter to set the image size.
Image(
painter = painterResource(id = R.drawable.cat),
contentDescription = "cat image",
modifier = Modifier.size(250.dp)
)
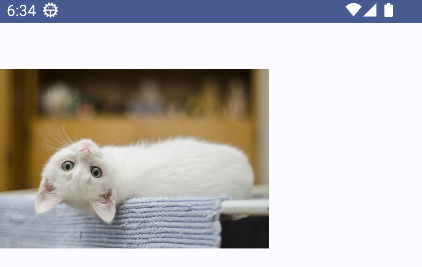
Image Shape
We can manipulate image shapes with Modifier.clip()
in the composable image.
Image(
painter = painterResource(id = R.drawable.cat),
contentDescription = "cat image",
modifier = Modifier.clip(CircleShape)
)
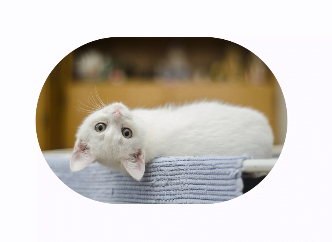
Note: In the Modifier.clip()
function, we can also pass RoundedCornerShape
function with dp
or pixel
as a parameter to manipulate the shape of the image.
In the above code, we use CircleShape
but the image isn’t. Now, we will use size
parameter of Modifier and contentScale
parameter of Image composable together with clip
parameter of the Modifier to make an image circle.
- Content Scale – It is an optional parameter of the image composable function used to determine the aspect ratio scaling to be used if the bounds are a different size from the intrinsic size of the provided Painter.
Image(
painter = painterResource(id = R.drawable.cat),
contentDescription = "cat image",
modifier = Modifier.clip(CircleShape).size(200.dp),
contentScale = ContentScale.Crop
)
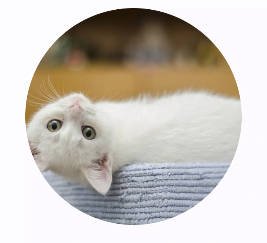
Border on Image in Jetpack Compose
We can provide a border to an image with the border extension function of Modifier. Remember, it takes three parameters width, color and shape. The shape parameter has a default value set to RectangleShape and if your image is in a circle shape then you should provide CircleShape
to border function as well otherwise border will not match the image. Let’s see the difference with an example:
Image(
painter = painterResource(id = R.drawable.cat),
contentDescription = "cat image",
modifier = Modifier
.clip(CircleShape)
.size(200.dp)
.border(4.dp, color = Color.Green),
contentScale = ContentScale.Crop
)
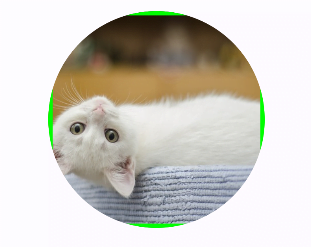
Image(
painter = painterResource(id = R.drawable.cat),
contentDescription = "cat image",
modifier = Modifier
.clip(CircleShape)
.size(200.dp)
.border(4.dp, color = Color.Green, shape = CircleShape),
contentScale = ContentScale.Crop
)
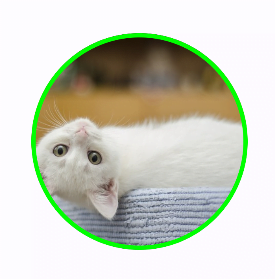
Now, we are left with two parameters of the image composable function. Let’s see them with examples
- Color Filter – With the help of a color filter, you can apply different tints to your painter.
- Alpha – It applies an optional opacity to the painter when it is rendered on the screen and its default value is completely opaque means fully visible. Its value range is 0.0 – 1.0 as a float.
Image(
painter = painterResource(id = R.drawable.ic_poke),
contentDescription = "pokemon image",
modifier = Modifier.size(200.dp),
colorFilter = ColorFilter.tint(Color.Green)
)
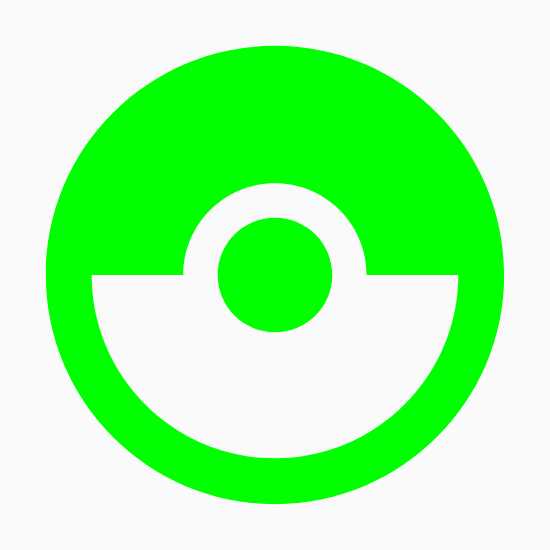
Image(
painter = painterResource(id = R.drawable.ic_poke),
contentDescription = "pokemon image",
modifier = Modifier.size(200.dp),
alpha = 0.4f,
colorFilter = ColorFilter.tint(Color.Blue)
)
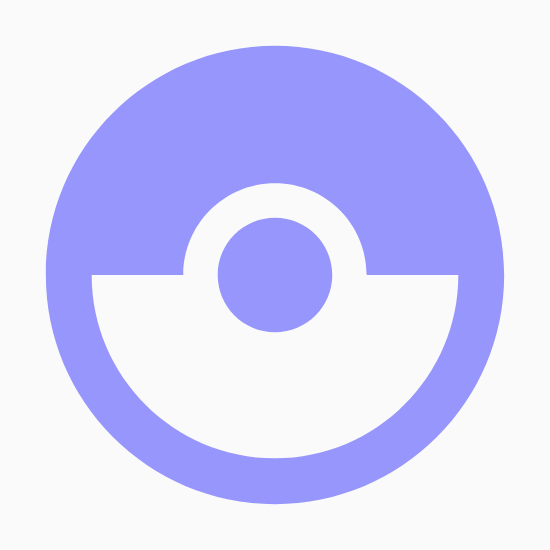
The image composable function is very important for any developer because we see images everywhere and without images no UI is complete. So practice on your own and if you get stuck then go to Android official documentation for reference. Thanks for visiting this post.
Happy Coding 🙂
2 thoughts on “Image in Jetpack Compose”